Automatically update if let and guard let for Swift 5.7
If you've seen the WWDC What's new in Swift? video, you've likely seen the updates to the if let
and guard let
syntax.
Prior to Swift 5.7, it was common to unwrap an Optional
variable and assign to a variable with the same name:
guard let self = self else {
return
}
if let model = model {
...
}
Fortunately, this syntax has been simplified in the latest version of Swift. We can now simply re-write these expressions like this:
guard let self else {
return
}
if let model {
...
}
While this is a welcome change, how can we update our entire codebase to reflect this new cleaner syntax? Regular expressions.
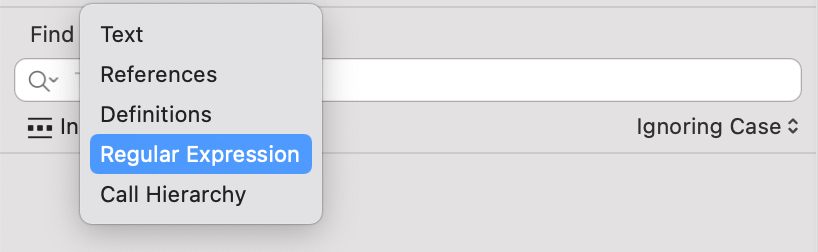
You can use the following regular expressions to perform this conversion across your entire codebase.
guard let
Find: guard let (\w+\b) = \b\1\s[else {]
Replace: guard let $1 else {
if let
Find: if let (\w+\b) = \b\1\s[{]
Replace: if let $1 {
This should convert all instances of guard let * = * else
and if let * = *
to new Swift 5.7 syntax π.
As a bonus, here's a script you can add to your pre-commit hooks to enforce this new convention:
import sys
import re
if __name__ == '__main__':
arguments = sys.argv
# The first parameter is always the call to this executable "python3 check-if-let-shorthand" which we can drop
arguments.pop(0)
# Searches through all of the modified files verifying the usage of the if-let and guard-let shorthand
for argument in arguments:
file = open(argument, "r")
text = file.read()
if_let_pattern = r"if let\s(\w+\b)\s=\s\b\1\s[{]"
guard_let_pattern = r"guard\slet\s(\w+\b)\s=\s\1\s(else)"
if len(re.findall(if_let_pattern, text)) > 0 or len(re.findall(guard_let_pattern, text)) > 0:
print("Invalid if-let or guard-let syntax in " + argument)
exit(1)
exit(0)
If you're interested in more articles about iOS Development & Swift, check out my YouTube channel or follow me on Twitter.
If you're an indie iOS developer, make sure to check out my newsletter - Indie Watch where I feature a new iOS developer and their personal projects every week.
Do you have an iOS Interview coming up?
Check out my book Ace The iOS Interview!